Migration, Seeder and Factory Creator In Nodejs
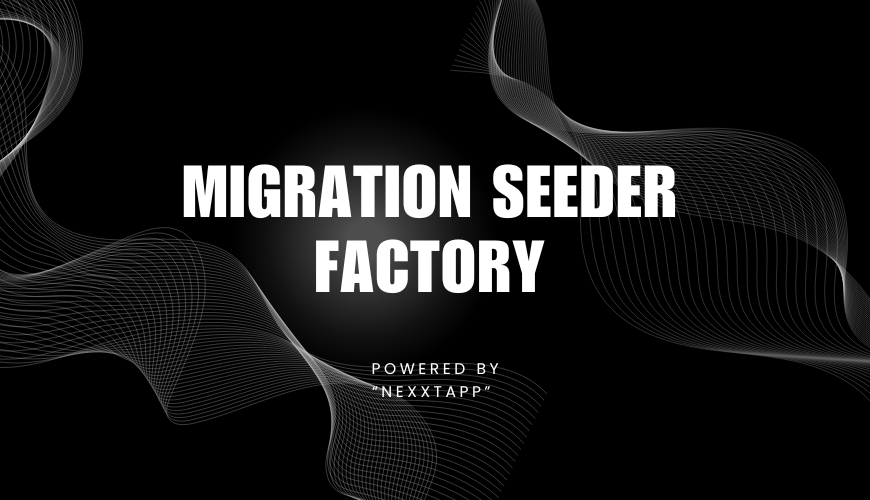
You can use the “migration-seeder-factory” package in Node.js to effortlessly generate migrations and seed data, even without extensive knowledge of database queries or data type syntax. Just follow the provided steps and syntax to begin.
Migration, Seeder, and Factory Creator
This package provides an easy way to generate migrations, seeders, and factories using built-in commands in Node.js. Developed by NexxtApp, this tool streamlines database management for your applications.
Note: MongoDB functionality will be available soon. Meanwhile, all features for MySQL and PostgreSQL are fully functional and ready to use.
Features
- Generate migrations, seeders, and factories effortlessly
- Simple CLI commands for quick setup
- Compatible with Node.js version v18.20.7 and later.
- Supports PostgreSQL, MySQL
Installation
npm install migration-seeder-factory
How to Use After Installation
Usage
Run the following commands to create respective database assets:
Environment variables required for database connection that must be set in your .env
file.
# Currently This Variable For MongoDB Not in Use, But Will Be Available Soon
MONGO_CONNECTION_URL=
MYSQL_HOST=
MYSQL_PORT=
MYSQL_USER=
MYSQL_PASSWORD=
MYSQL_DATABASE=
PG_HOST=
PG_PORT=
PG_USER=
PG_PASSWORD=
PG_DATABASE=
# postgres or mysql
DEFAULT_DB_TYPE=
Note: --db=
is optional. Set default database via DEFAULT_DB_TYPE
in .env
.
Commands
Create a Migration
MySQL:
npx nexxt make:migration create_sites_table --db=mysql
PostgreSQL:
npx nexxt make:migration create_sites_table --db=postgres
Run Migrations
MySQL (all files):
npx nexxt migrate --db=mysql
MySQL (specific file):
npx nexxt migrate 20250223154656_create_site_table --db=mysql
PostgreSQL (all files):
npx nexxt migrate --db=postgres
PostgreSQL (specific file):
npx nexxt migrate 20250223154656_create_site_table --db=postgres
Rollback Migrations
MySQL (all):
npx nexxt migrate:rollback --db=mysql
MySQL (specific file):
npx nexxt migrate:rollback create_sites_table --db=mysql
PostgreSQL (all):
npx nexxt migrate:rollback --db=postgres
PostgreSQL (specific file):
npx nexxt migrate:rollback create_sites_table --db=postgres
Create a Seeder
MySQL:
npx nexxt make:seeder sites_seeder --db=mysql
PostgreSQL:
npx nexxt make:seeder sites_seeder --db=postgres
Create a Factory
MySQL:
npx nexxt make:factory sites_factory
Run Seeders
MySQL (all):
npx nexxt seed --db=mysql
MySQL (specific file):
npx nexxt seed sites_seeder --db=mysql
PostgreSQL (all):
npx nexxt seed --db=postgres
PostgreSQL (specific file):
npx nexxt seed sites_seeder --db=postgres
Migration Object
Use this JSON structure to define migrations for MySQL and PostgreSQL:
{
"migrations": [
{
"action": "create",
"table": "sites",
"columns": [
{
"name": "id",
"type": "int",
"primaryKey": true,
"autoIncrement": true
},
{
"name": "name",
"type": "varchar(255)",
"unique": true,
"nullable": false
},
{
"name": "user_id",
"type": "int",
"unsigned": true //this should be false when you use mysql
},
{
"name": "age",
"type": "varchar(100)",
"nullable": false,
"default": 0
},
{
"name": "dob",
"type": "date",
"nullable": true
},
{
"name": "createdAt",
"type": "timestamp",
"default": {
"value": "CURRENT_TIMESTAMP"
}
},
{
"name": "updatedAt",
"type": "timestamp",
"default": {
"function": "ON_UPDATE_TIMESTAMP"
}
},
{
"name": "deletedAt",
"type": "timestamp",
"nullable": true
}
],
"indexes": [
{
"name": "idx_sites_name",
"columns": [
"name"
],
"unique": true
}
],
"foreignKeys": [
{
"name": "fk_sites_user",
"column": "user_id",
"referenceTable": "users",
"referenceColumn": "id"
}
],
"engine": "InnoDB",
"charset": "utf8mb4"
},
{
"action": "alter",
"table": "sites",
"changes": {
"add": [
{
"name": "email",
"type": "varchar(100)",
"nullable": true,
"after": "name"
},
{
"name": "owner_id",
"type": "int",
"nullable": true,
"unsigned": true
}
],
"modify": [
{
"name": "age",
"type": "int",
"nullable": false,
"after": "id"
}
],
"drop": [
"dob"
],
"addForeignKeys": [
{
"name": "fk_sites_owner",
"column": "owner_id",
"referenceTable": "users",
"referenceColumn": "id"
}
],
"dropForeignKeys": [
"fk_sites_user"
],
"dropIndex": [
"idx_sites_name"
]
}
}
],
"rollback": [
{
"action": "drop",
"table": "sites",
"dropIfExists": true,
"ignoreForeignAndCascade": true
}
]
}
This JSON object represents a structured way to define database migrations, compatible with both MySQL and PostgreSQL. Below is a breakdown of each key and its purpose in generating database queries:
Migration Keys
- The migrations array contains objects that define database schema changes.
Create Table (action: "create")
- Defines a new table (sites or whatever table name) with the following properties:
- columns: Specifies the columns in the table.
- name: Column name.
- type: Data type (e.g., int, varchar(255), timestamp).
- primaryKey: Marks the column as a primary key.
- autoIncrement: Enables auto-incrementing for primary keys.
- unique: Enforces unique constraint.
- nullable: Determines if the column can store NULL values.
- default: Assigns a default value to the column.
- unsigned: Ensures only positive values (MySQL-specific).
- indexes: Defines indexes for faster queries.
- name: Index name.
- foreignKeys: Defines relationships between tables.
- name: Foreign key constraint name.
- column: Column in the sites table that references another table.
- referenceTable: Table being referenced.
- referenceColumn: Column in the referenced table.
- engine: Specifies the storage engine (MySQL-specific, e.g., InnoDB).
- charset: Defines the character encoding (e.g., utf8mb4).
- after: Specifies whether a column is being modified or added during an ALTER operation Only. You can define its position by placing it after a specific existing column. (This work only with mysql database type)
- columns: Specifies the columns in the table.
Alter Table (action: "alter")
- Modifies the existing sites or whatever table name table:
- add: Adds new columns (email, owner_id).
- modify: Changes column properties (age changed to int).
- drop: Removes columns (dob).
- addForeignKeys: Adds new foreign keys.
- dropForeignKeys: Removes existing foreign keys.
- dropIndex: Drops existing indexes.
Rollback
- Defines how to revert changes if needed:
- action: "drop" — Drops the sites or whatever table name table.
- dropIfExists: Ensures the table is only dropped if it exists.
- ignoreForeignAndCascade: Ensures dependent records are removed properly.
Compatibility with MySQL & PostgreSQL
- Compatible with Both:
- Table creation, column definitions, and modifications.
- Indexing and foreign key constraints.
This structure allows for seamless database migrations across MySQL and PostgreSQL. 🚀
This Is for seeder and factory
In this context, "factory" refers to the factory name that you need to create. You must specify the file name you want to execute.
Seeder Object
{
"seed": [
{
"table": "sites",
"factory": "users_factory",
"execution_count": 2,
"createOrUpdate": {
"matchColumns": [
"name"
],
"operator": "or"
},
"custom": {
"query": "",
"execution_count": 0
}
},
{
"table": "sites",
"factory": "users_factory",
"execution_count": 0,
"createOrUpdate": {
"matchColumns": [
"name",
"status"
],
"operator": "and"
},
"custom": {
"query": "INSERT INTO space_types (name, status) VALUES ('Test Name', 1)",
"execution_count": 1
},
"referenceQuery": {
"query": "INSERT INTO log (name, context) VALUES ('{forRefName}', '{context}_alias')",
"columns" : {
"forRefName" : "name",
"context": "context_id"
}
}
}
]
}
Keys and Their Uses
seed (Array)
- The seed array contains multiple objects, each specifying how data should be inserted into a particular table.
Each Seed Object Contains:
- table (String): The name of the table where data will be inserted.
- Example:
"table": "sites"
- Example:
- factory (String): Specifies the factory name used to generate data automatically.
- Example:
"factory": "users_factory"
- Example:
- execution_count (Integer): Determines how many times the factory should generate and insert data into the table.
2
→ Runs the factory twice, inserting two records.0
→ Factory-based seeding is disabled for this entry.
- createOrUpdate (Object): Logic for checking whether a record already exists in the table before inserting or updating.
matchColumns
: Columns used for matching existing records.operator
: "or" / "and" between conditions.
- custom (Object): Raw SQL query execution.
query
(String): SQL statement.execution_count
(Number): How many times to run the custom query.
- referenceQuery (Object): Insert data into another table using values from the current row.
query
: SQL with placeholders ({}
).columns
: Maps placeholders to current table columns.
Factory Object
This defines the column information that you want to modify or affect.
{
"columns": [
{
"context_id": {
"fake": false,
"type": "number",
"custom": {
"reference_table": {
"table": "sites",
"column": "id"
}
}
},
"first_name": {
"fake": false,
"type": "",
"custom": "{name}",
"convert_case" : "lower"
},
"name": {
"fake": true,
"type": "string",
"custom": "",
"manipulation": {
"regex" : "\\s",
"replace_with" : "-"
}
},
"father_name": {
"fake": true,
"type": "string",
"custom": "",
"convert_case" : "lower"
},
"status": {
"fake": false,
"type": "",
"custom": 1
}
}
]
}
Object Description for Column Data Generation
This JSON object defines how column data should be generated for seeding purposes. It supports both fake (randomly generated) values and custom static values.
Keys and Their Uses
- columns (Array): The columns array contains objects that define how each column's data should be populated.
- Each Column Object Contains:
- column_name (Object): The key represents the column name in the database.
- fake (Boolean):
true
for Faker-generated values;false
for manual. - type (String): Data type (
string
,number
,boolean
,date
, etc.). - custom: Fixed value or
{column_name}
for self-reference. - convert_case:
"lower"
,"upper"
, or"capitalize"
. - manipulation: Regex-based modifications.
- fake (Boolean):
- column_name (Object): The key represents the column name in the database.
Supported Types
Here are all the supported types:
number
smallint
mediumint
bigint
tinyint
float
double
decimal
boolean
uuid
string
char
varchar
longtext
alphanumeric
email
url
username
password
phone
address
city
state
country
zip
ipv4
ipv6
mac_address
company
company_suffix
color
hexcolor
date
time
datetime
future_date
past_date
credit_card
currency
iban
bic
job_title
Custom (String | Number
, etc.)
- When
fake
is set tofalse
, this field specifies a fixed value for the column.
Custom Object for Foreign Key
- For example, in the
context_id
(or any designated column) field, instead of manually setting a number or generating a fake value, you can use an object with areference_table
key. - This instructs the seeder to look up a value from another table.
- In this case, it will retrieve an
id
from thesites
table.
Maintaining Referential Integrity
- This ensures that the value inserted into the
context_id
(or any designated column) is a valid existing key from thesites
table. - It’s especially useful for foreign key relationships, as it prevents hardcoding or guessing values.
Different Behavior for Each Field (Example: context_id
)
fake: false
– No fake value is generated.custom
– The value is obtained by referencing the actualid
from thesites
table.
Custom for Self Table Column Value ({column_name}
)
- The
custom
key is used to assign a fixed or computed value to a column instead of generating a fake one. - When you set a value like
{name}
, you’re using a self-reference marker. - This tells the seeder logic that the value for this column should come from the already-generated (or provided) value of another column — in this case, the
"name"
column.
Marker Detection
- When the seeder processes the factory, it checks if the
custom
value matches a pattern like{other_column}
. - If it does, the value isn’t manipulated (e.g., by fake data or regex) but is saved as a marker.
Convert Case ("convert_case": "lower"
)
- This key help to column convert value into lower/upper/capitalize case.
Manipulation
- In this configuration, the
manipulation
property is used to modify the column's value using regex.
Regex Pattern
"regex": "\\s"
Matches any whitespace character in the string.
Replacement String
"replace_with": "-"
Replaces matches (e.g., spaces) with hyphens.
Purpose in Context
- For the
name
column, even if the value is generated or custom, it will be post-processed to replace spaces with hyphens. - Example:
"John Doe"
becomes"John-Doe"
.
Note:
This is not the final release — new features will be added in future versions!
Stay updated by visiting NextApp, creating an account, and exploring our useful products and services. 🚀
Simplify Your Database Workflow
Manage Migrations & Seeders with Ease
Say goodbye to repetitive database tasks. With the `migration-seeder-factory` package, you can easily generate migrations, seeders, and factories without needing deep SQL or ORM knowledge. It’s perfect for fast prototyping, testing, and scaling your Node.js applications with minimal setup. Built for developers who want speed, structure, and flexibility.
Quickly scaffold your database logic using a powerful and intuitive system. Reduce boilerplate, ensure referential integrity, and customize value generation with ease — all while staying in your Node.js environment.